How to Use Class Inheritance in Python
By Birtchum Thompson | April 4, 2020
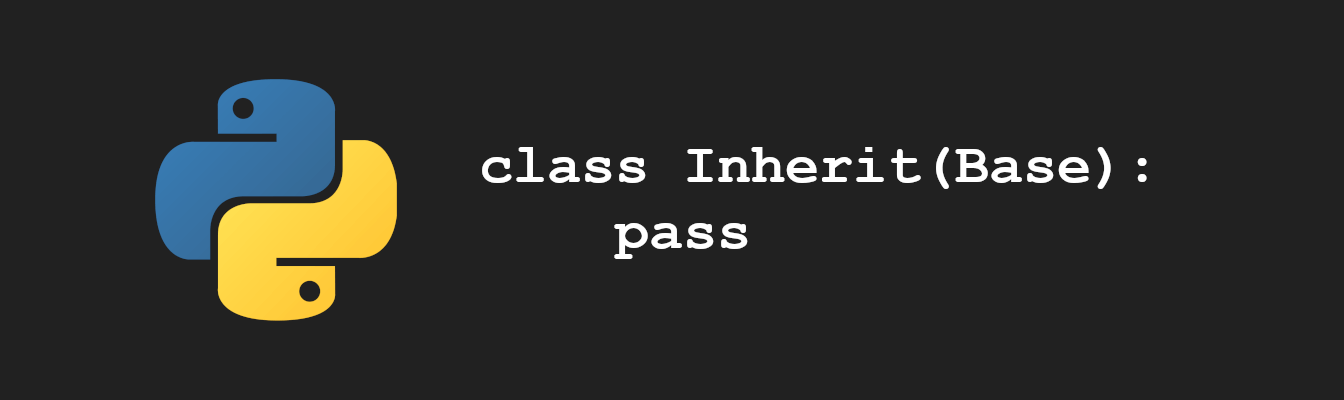
Class inheritance is an important concept in Python and OOP languages in general. Inheritance’s main benefit is reusability, allowing the properties and methods of the parent class to be used by the child class.
We will start by creating our parent class, Vehicle. The Vehicle class’s constructor will accept 3 arguments: type, wheels, and engine. Wheels and engine are initialized as True since we can assume that all vehicle types we will deal with in this tutorial have both. The Vehicle class also has a single method that prints a sentence revealing the vehicle type.
To test our Vehicle class, we create an instance and print each property, then call the get_type() method like this:
Then, run the application. The output prints the vehicle’s properties and vehicle type sentence as expected.
train True True This vehicle is a train
Next, we remove our tests and create 2 child classes that will inherit from Vehicle: Car and Truck. For inheritance to work, Vehicle must be passed as an argument to both Car and Truck. The child classes also each accept 2 additional arguments: brand and color. We can initialize the vehicle types for our child classes since we know that they are car and truck, respectively. Our child class constructor will assign brand and color as object attributes, but the super() function must be used to finish initialization with the parent class to be able to access its properties and methods.
Let’s test the application by creating instances of both car and truck, then printing out all of their properties and their get_type() method.
After running the application, we see that our child classes did, indeed, inherit the properties and method of their parent class.
Dodge red truck True This vehicle is a truck Chevy blue car True This vehicle is a car
Join the Discussion
Also Read
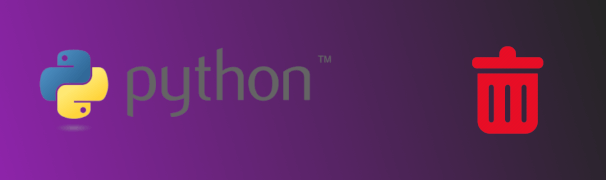
Create a Python script to delete files older than x days.
Read Now!