How to Use Class Methods in Python
By Birtchum Thompson | April 6, 2020
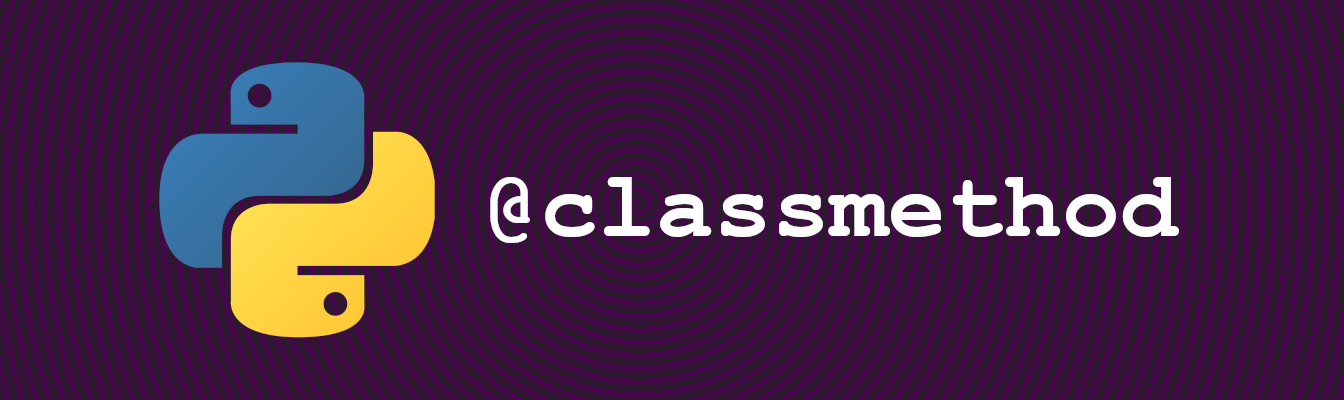
Python’s class methods provide a way to create alternate constructors for a given class. This is especially useful if the data used to instantiate the class objects comes from varying source formats like typical keyboard input, JSON, and CSV.
In this example, we define a Pizza class that accepts 5 arguments: size, toppings, dough, sauce, and cheese. The default value True is assigned to dough, cheese, and sauce, so only size and toppings must be passed to the Pizza class when instantiating an object. If a Pizza object is created, its properties are available and can be printed as shown below.
But what if there is a need to create Pizza objects from JSON input instead? That is where Python’s class methods and the @classmethod decorator come in. In the example below, there is a new method named from_json. It is using the @classmethod decorator and accepts 2 arguments: the Pizza class itself, and some JSON data. Standard convention is to use cls when passing the class to the method, but it can be anything except for keywords like ‘class’, of course. It is also standard convention to name class methods with ‘from’ and the source format that will be used to instantiate the object (from_csv, from_user_input, etc.).
Finally, the JSON data is parsed before creating the object. The JSON data only contains size and toppings because the default values of True for dough, sauce, and cheese will still be assigned when the object is created. To instantiate the new Pizza object with the parsed JSON values, the size and toppings are simply passed to the class (cls) and the resulting Pizza instance is returned.
Join the Discussion
Also Read
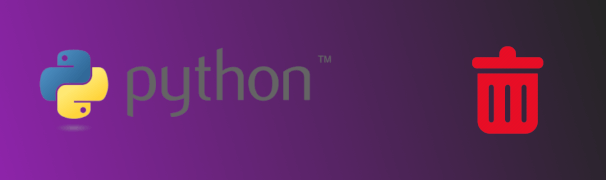
Create a Python script to delete files older than x days.
Read Now!