How to Use Class Inheritance in JavaScript
By Birtchum Thompson | April 7, 2020
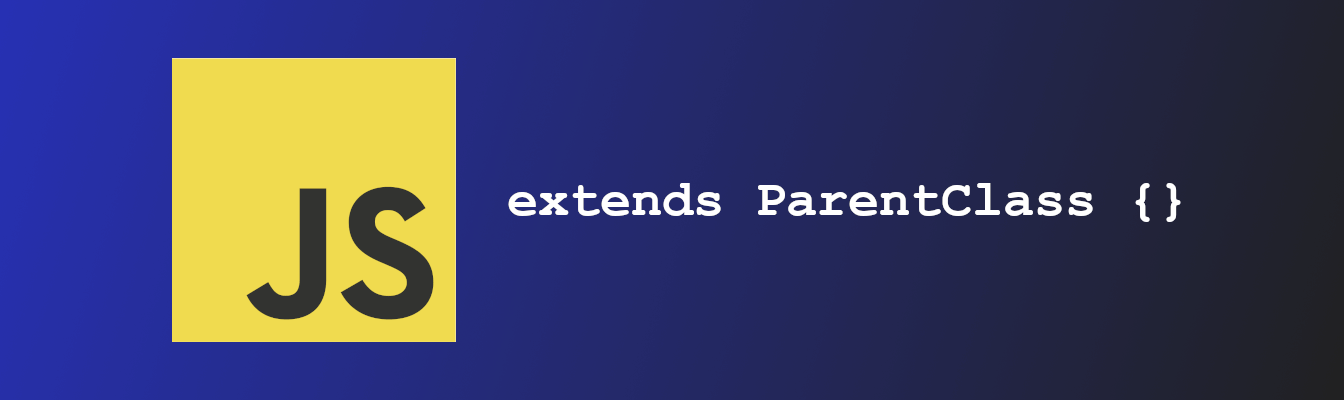
Class inheritance allows a child class to extend a parent class. Class inheritance promotes reusability by allowing the developer to write less code. This tutorial will demonstrate how to use class inheritance in vanilla JavaScript.
First, the base class is defined. In this example, the base class is Guitar, from which the BassGuitar and LeadGuitar classes inherit from. The Guitar class accepts 2 arguments: brand and color. It also contains 2 functions. The first is play which accepts a tempo argument and prints a sentence to the console stating the tempo that the guitar is playing at. The second function, tune, accepts a key argument and prints a sentence to the console stating the key that the guitar is tuning to.
Next, the child classes BassGuitar and LeadGuitar are added. The ‘extends’ keyword is used to properly inherit from the Guitar parent class. In addition to accepting the Guitar base class arguments, the BassGuitar class accepts a string count argument and the LeadGuitar class accepts a volume argument. Within the constructors for the child classes, the super function must be called first to initialize the object with the base class, then the child class properties can be assigned. Each child class also has a unique function. The BassGuitar class has a slap function that accepts a style argument, while the LeadGuitar class features a shred function that also accepts a style argument.
To test the new program, an object from each class is created and the properties and function results of each are printed to the console.
Output:
Fender blue The Fender guitar is playing at 125 BPM. The Fender guitar is tuning to A minor Ernie Ball red 5 The Ernie Ball guitar is playing at 150 BPM. The Ernie Ball guitar is tuning to E# major The Ernie Ball bass is being slapped funk style! PRS purple 11 The PRS guitar is playing at 150 BPM. The PRS guitar is tuning to D minor The PRS lead guitar is being shredded metal style!