How to Use the Argparse Module in Python
By Birtchum Thompson | April 12, 2020
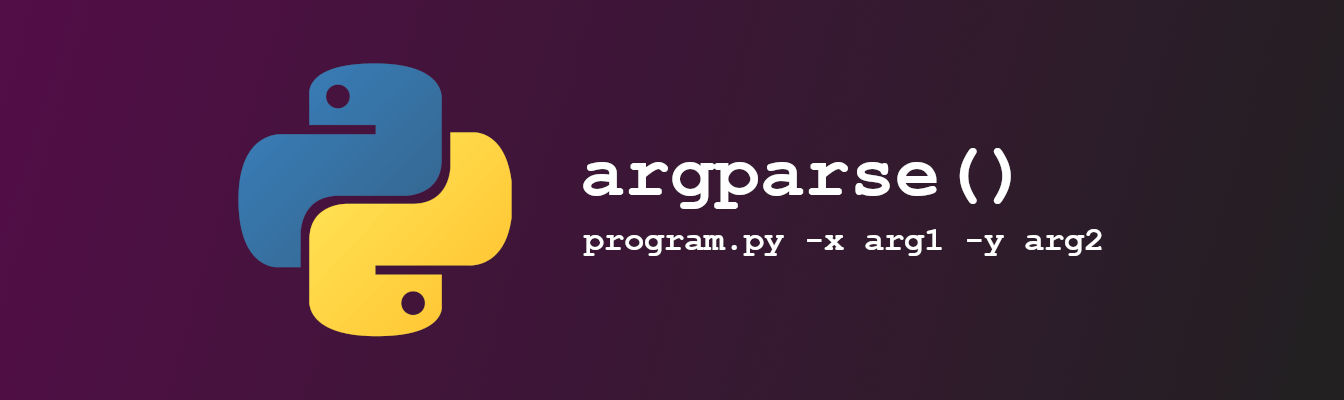
The argparse module for Python enables a CLI program to accept command line arguments when executing a program. Argparse provides support for both positional and optional arguments while supplying enough configuration options to make scripting with argparse a breeze.
Before using argparse, it must be installed in the environment. Enter the following shell command to install argparse.
With argparse installed, we can begin scripting our program. First, import the argparse module and set up the main structure of the program. We create a variable named args that will hold the parsed arguments that are returned from the parse_args function. Within the parse_args function, a parser object is created with a few configuration options. All of the options we are using are displayed when running the program with the -h or --help flags, which displays a message with instructions on how to use our program.
The first option, prog, defines how to execute our program from the command line and is displayed in the usage section of the help message. The second option, description, is simply a description of the program. The description is displayed directly beneath the usage info in the help message. Finally, the epilog option is used to provide any additional information about the program and is displayed at the end of the help message.
Now, we will add a required positional argument and a required optional argument. The positional argument will capture the math operation to be performed while the required optional argument will capture the 2 numbers to be used in the calculation. The positional argument will be named operation and this name will be used later to access its value. We then provide a list of valid math operation choices. Our program will add, subtract, multiply, and divide so those are added to the choices list. Finally, the help option describes what the argument’s purpose is and is displayed along with any corresponding flags between the description and epilog portions of the help message. Our required optional argument is named numbers and is invoked by either the -n or --numbers flag. A help message is provided for this argument just like the positional argument. For this argument, a few additional options are provided, however. Since this is a math program, we will want to convert all numeric input to float type, which is achieved by setting the type option equal to float. Then, the nargs option is set equal to 2. The nargs option defines the number of arguments that will be expected after the flag. In this case it is 2. After nargs, the metavar option is declared. The metavar option defines how our arguments are displayed in the help message. The values NUM1 and NUM2 will be used to make the usage information easy to understand. Lastly, required is set to True because the program must receive numeric data to perform operations on.
With some arguments defined for argparse, we can check out our help message now. To do so, execute the following shell command, replacing program.py with the name of your Python file.
Output:
usage: pipenv run python program.py [-h] -n NUM1 NUM2 [-l | -s] {add,subtract,multiply,divide} Simple math program positional arguments: {add,subtract,multiply,divide} The math operation to be performed optional arguments: -h, --help show this help message and exit -n NUM1 NUM2, --numbers NUM1 NUM2 Have fun with math!
Nice! It looks like a legitimate help message with minimal effort on our part. Now we will add 2 more arguments, but both will be optional. These arguments will simply format the result of the math calculation as strings: one as a short string, the other as a long string. We are doing something different with these options though: we are making them mutually exclusive. Mutually exclusive simply means that an error will be raised if both options are used at the same time. Mutual exclusivity is achieved by creating an exclusive group and adding the arguments to the group instead of the parser object itself. Both arguments still receive the help option and message, but we encounter a new option: action. The action variable controls what value is stored when the flag is used. With the numbers argument from above, the default action is to store whatever values are entered after the flag. In this case, however, we simply want to store a value of True if the flag is used, so the action option is set equal to store_true.
Let’s take another look at our help message to make sure our new arguments are correctly displayed.
Output:
usage: pipenv run python program.py [-h] -n NUM1 NUM2 [-l | -s] {add,subtract,multiply,divide} Simple math program positional arguments: {add,subtract,multiply,divide} The math operation to be performed optional arguments: -h, --help show this help message and exit -n NUM1 NUM2, --numbers NUM1 NUM2 The numbers for the operation -l, --long-string Prints the answer in a long string -s, --short-string Prints the answer in a short string Have fun with math!
All good! Now let’s add our simple math calculation functions.
All that’s left to do now is some basic logic to determine what function to call and how to print the answer. We create the print_answer function first. This function receives 2 arguments: the actual arguments supplied when the program was executed and the answer to the math operation. Within the function, it is determined whether the long_string or short_string option was provided, or neither, then prints the answer accordingly. Finally the control-flow logic is added beneath our args variable. The logic determines which math operation is desired, calls the appropriate function, and sends the result to the print_answer function.
Finally, its time to test our program. Enter the following shell command to test addition.
4.0
The answer you're looking for is 4.0
The answer is 4.0
Subtraction:
2.0
The answer you're looking for is 2.0
The answer is 2.0
Multiplication:
4.0
The answer you're looking for is 4.0
The answer is 4.0
And Division:
2.0
The answer you're looking for is 2.0
The answer is 2.0
Join the Discussion
Also Read
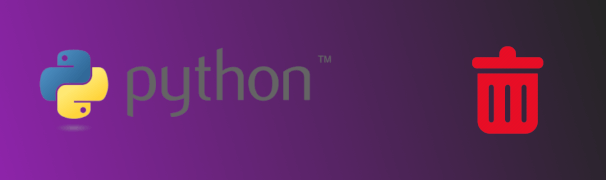
Create a Python script to delete files older than x days.
Read Now!