How to Sort a List of Objects by an Object Attribute in Python
By Birtchum Thompson | April 12, 2020
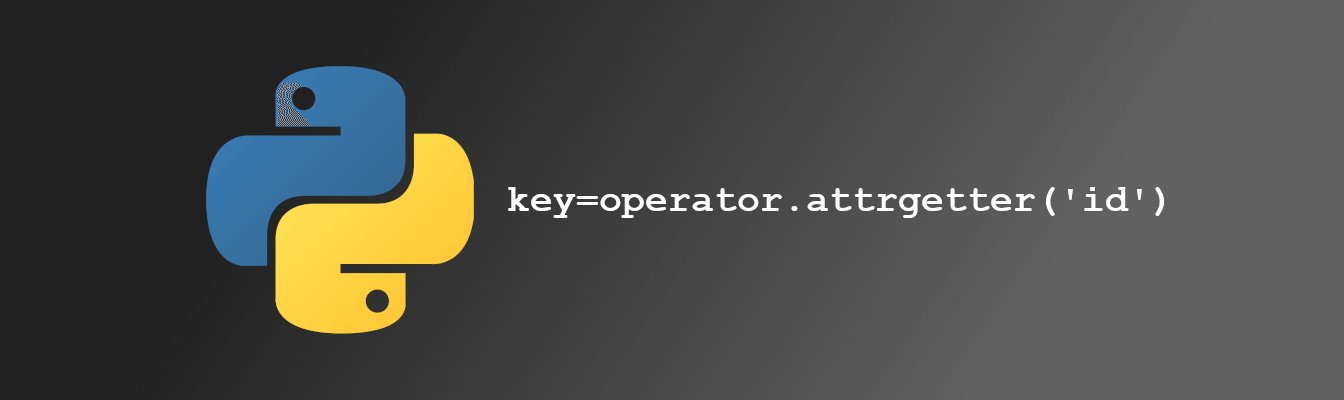
In some cases it is useful to sort a list of objects alphabetically or by an attribute like an ID number. The Python operator module allows us to easily achieve this.
First, import the operator module and create the Bird class. The Bird class will accept 2 arguments: kind and wings. Wings is set to True by default meaning we will not have to pass this value when instantiating Bird objects.
Next, we create our sort function and control-flow logic. The sort_alphabetically function will receive a list of Bird objects and a reverse directive, which is set to False by default. The Bird objects are then sorted alphabetically by kind. The control-flow logic contains a list of bird kinds and a list of Bird objects created from the bird kinds list.
Finally, we can start testing the program. First, we will print out the list of bird objects before sorting as a reference point by adding the following for-loop to our program.
Output:
robbin canary seagull peacock
Then we sort the Bird objects alphabetically and print their kinds.
Output:
canary peacock robbin seagull
Lastly, we sort in reverse-alphabetical order and print the Bird object kinds.
Output:
seagull robbin peacock canary